Basic Drawing Functions - Rectangles, Ellipses, Line Styles
Ex.1 Draw some boxes while changing colors
Sub ColorBox()
Dim c, myBoxSize, myInterval
Dim x1, y1, x2, y2
InitializeGraphics ' Declaration of using graphics library
myBoxSize = 22
myInterval = myBoxSize + 5
x1 = 0
y1 = 10
For c = 0 To 14
DrawRectangle x1, y1, x1 + myBoxSize, y1 + myBoxSize, QBColor(c) ' Draw a box
x1 = x1 + myInterval
Next c
x1 = 0
y1 = y1 + myInterval
For c = 0 To 14
DrawRectangleFill x1, y1, x1 + myBoxSize, y1 + myBoxSize, QBColor(c) ' Draw a filled box
x1 = x1 + myInterval
Next c
x1 = 0
y1 = y1 + myInterval
For c = 0 To 14
' Draw a box with different fill color and line color
DrawRectangleFill x1, y1, x1 + myBoxSize, y1 + myBoxSize, QBColor(c), QBColor(c + 1)
x1 = x1 + myInterval
Next c
End Sub
Ex.2 Draw some circles while changing colors
Sub ColorCircle()
Dim i, c, r, d
Dim x, y
r = 20
d = 50
InitializeGraphics ' Declaration of using graphics library
c = 0 ' Initialize color number
For i = 1 To 2
For j = 1 To 8
DrawOval x, y, r, r, QBColor(c) ' Draw a circle
c = c + 1
x = x + d
Next j
y = y + d
x = 10
Next i
c = 0
For i = 1 To 2
For j = 1 To 8
DrawOvalFill x, y, r, r, QBColor(c) ' Draw a filled circle
c = c + 1
x = x + d
Next j
y = y + d
x = 10
Next i
End Sub
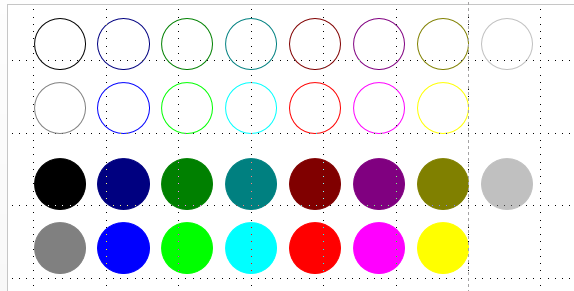
Ex.3 Draw an ellipse
Sub DrawOvalTest()
InitializeGraphics ' Declaration of using graphics library
y = 300
' Draw an ellipse
DrawOval 70, y, 70, 30, vbGreen
' Draw a circle
DrawOval 190, y, 20, 20, vbBlack
' Specification of radius ratio of oval and line color by default
DrawOval 240, y, 45
' Specification of line and fill color by QBColor function
DrawOvalFill 340, y, 35, , QBColor(9), QBColor(12)
End Sub
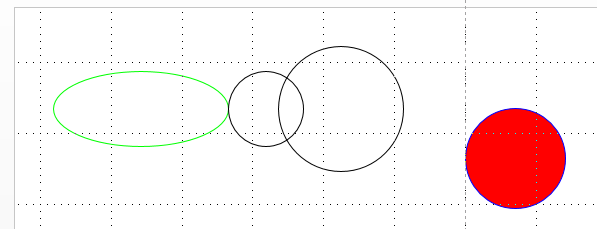
Ex.4 Change the line width and type
Sub LineStyleTest()
Dim c, y
Const x1 = 10
Const x2 = 310
Const d = 18
InitializeGraphics ' Declaration of using graphics library
gLineWidth = 6# ' Set the line width
c = QBColor(0)
y = 360
DrawLine x1, y, x2, y, c ' Draw a line
y = y + d
SetDashStyle msoLineDash ' Set line style
DrawLine x1, y, x2, y, c
y = y + d
SetDashStyle msoLineDashDot
DrawLine x1, y, x2, y, c
y = y + d
SetDashStyle msoLineDashDotDot
DrawLine x1, y, x2, y, c
y = y + d
SetDashStyle msoLineRoundDot
DrawLine x1, y, x2, y, c
y = y + d
SetDashStyle msoLineSquareDot
DrawLine x1, y, x2, y, c
y = y + d
SetDashStyle msoLineSolid ' Set the style of line - Solid line
DrawLine x1, y, x2, y, c
gLineWidth = 10# ' Set the thickness of line
y = y + d * 2
SetLineStyle msoLineSingle
DrawLine x1, y, x2, y, c
y = y + d
SetLineStyle msoLineThinThin
DrawLine x1, y, x2, y, c
y = y + d
SetLineStyle msoLineThinThick
DrawLine x1, y, x2, y, c
y = y + d
SetLineStyle msoLineThickThin
DrawLine x1, y, x2, y, c
y = y + d
SetLineStyle msoLineThickBetweenThin
DrawLine x1, y, x2, y, c
End Sub
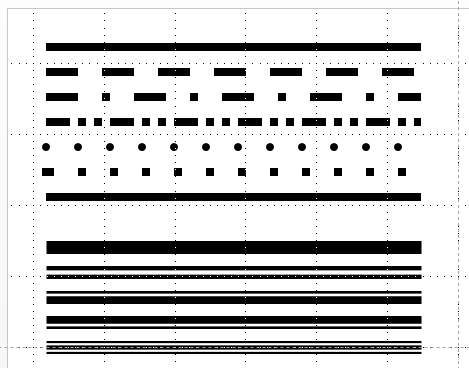
Sub ColorBox() Dim c, myBoxSize, myInterval Dim x1, y1, x2, y2 InitializeGraphics ' Declaration of using graphics library myBoxSize = 22 myInterval = myBoxSize + 5 x1 = 0 y1 = 10 For c = 0 To 14 DrawRectangle x1, y1, x1 + myBoxSize, y1 + myBoxSize, QBColor(c) ' Draw a box x1 = x1 + myInterval Next c x1 = 0 y1 = y1 + myInterval For c = 0 To 14 DrawRectangleFill x1, y1, x1 + myBoxSize, y1 + myBoxSize, QBColor(c) ' Draw a filled box x1 = x1 + myInterval Next c x1 = 0 y1 = y1 + myInterval For c = 0 To 14 ' Draw a box with different fill color and line color DrawRectangleFill x1, y1, x1 + myBoxSize, y1 + myBoxSize, QBColor(c), QBColor(c + 1) x1 = x1 + myInterval Next c End Sub
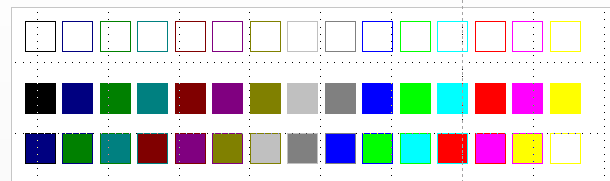
Sub ColorCircle() Dim i, c, r, d Dim x, y r = 20 d = 50 InitializeGraphics ' Declaration of using graphics library c = 0 ' Initialize color number For i = 1 To 2 For j = 1 To 8 DrawOval x, y, r, r, QBColor(c) ' Draw a circle c = c + 1 x = x + d Next j y = y + d x = 10 Next i c = 0 For i = 1 To 2 For j = 1 To 8 DrawOvalFill x, y, r, r, QBColor(c) ' Draw a filled circle c = c + 1 x = x + d Next j y = y + d x = 10 Next i End Sub
Ex.3 Draw an ellipse
Sub DrawOvalTest()
InitializeGraphics ' Declaration of using graphics library
y = 300
' Draw an ellipse
DrawOval 70, y, 70, 30, vbGreen
' Draw a circle
DrawOval 190, y, 20, 20, vbBlack
' Specification of radius ratio of oval and line color by default
DrawOval 240, y, 45
' Specification of line and fill color by QBColor function
DrawOvalFill 340, y, 35, , QBColor(9), QBColor(12)
End Sub
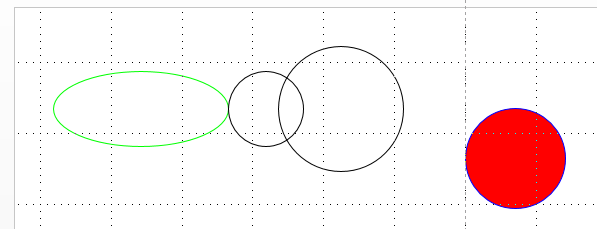
Ex.4 Change the line width and type
Sub LineStyleTest()
Dim c, y
Const x1 = 10
Const x2 = 310
Const d = 18
InitializeGraphics ' Declaration of using graphics library
gLineWidth = 6# ' Set the line width
c = QBColor(0)
y = 360
DrawLine x1, y, x2, y, c ' Draw a line
y = y + d
SetDashStyle msoLineDash ' Set line style
DrawLine x1, y, x2, y, c
y = y + d
SetDashStyle msoLineDashDot
DrawLine x1, y, x2, y, c
y = y + d
SetDashStyle msoLineDashDotDot
DrawLine x1, y, x2, y, c
y = y + d
SetDashStyle msoLineRoundDot
DrawLine x1, y, x2, y, c
y = y + d
SetDashStyle msoLineSquareDot
DrawLine x1, y, x2, y, c
y = y + d
SetDashStyle msoLineSolid ' Set the style of line - Solid line
DrawLine x1, y, x2, y, c
gLineWidth = 10# ' Set the thickness of line
y = y + d * 2
SetLineStyle msoLineSingle
DrawLine x1, y, x2, y, c
y = y + d
SetLineStyle msoLineThinThin
DrawLine x1, y, x2, y, c
y = y + d
SetLineStyle msoLineThinThick
DrawLine x1, y, x2, y, c
y = y + d
SetLineStyle msoLineThickThin
DrawLine x1, y, x2, y, c
y = y + d
SetLineStyle msoLineThickBetweenThin
DrawLine x1, y, x2, y, c
End Sub
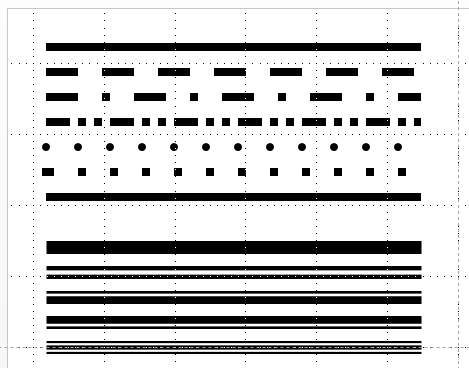
Sub DrawOvalTest() InitializeGraphics ' Declaration of using graphics library y = 300 ' Draw an ellipse DrawOval 70, y, 70, 30, vbGreen ' Draw a circle DrawOval 190, y, 20, 20, vbBlack ' Specification of radius ratio of oval and line color by default DrawOval 240, y, 45 ' Specification of line and fill color by QBColor function DrawOvalFill 340, y, 35, , QBColor(9), QBColor(12) End Sub
Sub LineStyleTest() Dim c, y Const x1 = 10 Const x2 = 310 Const d = 18 InitializeGraphics ' Declaration of using graphics library gLineWidth = 6# ' Set the line width c = QBColor(0) y = 360 DrawLine x1, y, x2, y, c ' Draw a line y = y + d SetDashStyle msoLineDash ' Set line style DrawLine x1, y, x2, y, c y = y + d SetDashStyle msoLineDashDot DrawLine x1, y, x2, y, c y = y + d SetDashStyle msoLineDashDotDot DrawLine x1, y, x2, y, c y = y + d SetDashStyle msoLineRoundDot DrawLine x1, y, x2, y, c y = y + d SetDashStyle msoLineSquareDot DrawLine x1, y, x2, y, c y = y + d SetDashStyle msoLineSolid ' Set the style of line - Solid line DrawLine x1, y, x2, y, c gLineWidth = 10# ' Set the thickness of line y = y + d * 2 SetLineStyle msoLineSingle DrawLine x1, y, x2, y, c y = y + d SetLineStyle msoLineThinThin DrawLine x1, y, x2, y, c y = y + d SetLineStyle msoLineThinThick DrawLine x1, y, x2, y, c y = y + d SetLineStyle msoLineThickThin DrawLine x1, y, x2, y, c y = y + d SetLineStyle msoLineThickBetweenThin DrawLine x1, y, x2, y, c End Sub